正在载入交互式动画窗口请稍等
压缩存储-对称矩阵 可视化交互式动画版
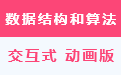
给定一个 下三角矩阵 Mat[][] ,任务是使用行主映射来存储矩阵。
下三角矩阵: 下 三角矩阵 是一个方阵,其中矩阵的下三角部分由非零元素组成,上三角部分由 0 组成。 二维矩阵 Mat[][] 的下 三角矩阵 在数学上定义为:
- 如果 i < j ,则设置 Mat[i][j] = 0 。
- 如果 i >= j ,则设置 Mat[i][j] > 0 。
说明: 下面是一个 5×5 的下三角矩阵。 一般来说,这样的矩阵可以存储在 二维数组 中,但是当涉及到大尺寸的矩阵时,它不是一个好的选择,因为由于存储了不需要的 0 而导致内存消耗很高。
这样的矩阵可以以优化的方式实现。
存储 大小为 N的 下三角矩阵 的有效方法:
- 非零元素的计数 = 1 + 2 + 3 + … + N = N * (N + 1) /2 。
- 0 的计数 = N 2 – (N * (N + 1) /2 = (N * (N – 1)/2 。
现在让我们看看如何在程序中表示下三角矩阵。 请注意,必须避免 存储 0 ,以减少内存消耗。 经计算,存储非零元素 需要 N*(N+1)/2空间。 以上面的例子为例, N = 5 。 需要大小为 5 * (5 + 1)/2 = 15 的 数组来存储非零元素。
现在,二维矩阵的元素可以逐行存储在一维数组中,如下所示:
除了将元素存储在数组中之外,还需要一个提取行号和列号对应的元素的过程。
使用
行主映射来存储下三角矩阵,索引
Mat[i][j]
处的元素
可以表示为:
数组 A[] 中 Mat[i][j] 矩阵的索引= [i*(i – 1)/2 + j – 1]
下面是上述方法的实现:
- C++
- C
- Java
- Python3
- C#
- JavaScript
C++
// C++ program for the above approach #include <bits/stdc++.h> using namespace std; // Dimensions of a matrix
static int N = 5; // Structure of the efficient matrix class Matrix { public : int * A; int size; }; // Function to set the
// values in the Matrix
void Set(Matrix mat, int i, int j, int x)
{ if (i >= j) mat.A[i * (i - 1) / 2 + j - 1] = x; } // Function to store the
// values in the Matrix
int Get(Matrix mat, int i, int j)
{ if (i >= j) return mat.A[i * (i - 1) / 2 + j - 1]; return 0; } // Function to display the
// elements of the matrix
void Display(Matrix mat) { int i, j; // Traverse the matrix for (i = 1; i <= mat.size; i++) {
for (j = 1; j <= mat.size; j++) {
if (i >= j) cout << mat.A[i * (i - 1) / 2 + j - 1] << " " ;
else cout << 0 << " " ; } cout << endl; } } // Function to generate an efficient matrix Matrix createMat(vector<vector< int > >& Mat) { // Declare efficient Matrix Matrix mat; // Initialize the Matrix mat.size = N; mat.A = new int [(mat.size * (mat.size + 1)) / 2];
int i, j; // Set the values in matrix for (i = 1; i <= mat.size; i++)
for (j = 1; j <= mat.size; j++)
Set(mat, i, j, Mat[i - 1][j - 1]); // Return the matrix return mat; } // Driver Code int main() { vector<vector< int > > Mat = { { 1, 0, 0, 0, 0 }, { 1, 2, 0, 0, 0 }, { 1, 2, 3, 0, 0 }, { 1, 2, 3, 4, 0 }, { 1, 2, 3, 4, 5 } }; // Stores the efficient matrix Matrix mat = createMat(Mat);
// Print the Matrix Display(mat); return 0; } // This code is contributed by Tapesh (tapeshdua420) |
C
Java
Python3
C#
JavaScript
1 0 0 0 0 1 2 0 0 0 1 2 3 0 0 1 2 3 4 0 1 2 3 4 5
时间复杂度:
O(N
2
)
辅助空间:
O(N
2
)